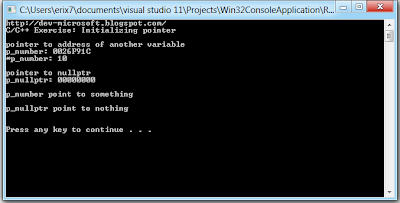
#include <iostream> #include <conio.h> using std::cin; using std::cout; using std::endl; int main(){ cout << "http://dev-microsoft.blogspot.com/" << endl; cout << "C/C++ Exercise: Initializing pointer" << endl << endl; int number = 10; int *p_number = &number; cout << "pointer to address of another variable" << endl; cout << "p_number: " << p_number << endl; cout << "*p_number: " << *p_number << endl; cout << endl; int *p_nullptr = nullptr; cout << "pointer to nullptr" << endl; cout << "p_nullptr: " << p_nullptr << endl; //cout << "*p_nullptr: " << *p_nullptr << endl; // <- Invalid /* Will cause Unhandled exception at 0x003F13AE in Win32ConsoleApplication.exe: * 0xC0000005: Access violation reading location 0x00000000. */ cout << endl; //Check if pointer point to anything if(p_number){ cout << "p_number point to something" << endl; }else{ cout << "p_number point to nothing" << endl; } cout << endl; if(p_nullptr){ cout << "p_nullptr point to something" << endl; }else{ cout << "p_nullptr point to nothing" << endl; } cout << endl; cout << endl; system("PAUSE"); return 0; }
nullptr represents a null pointer value. Use a null pointer value to indicate that an object handle, interior pointer, or native pointer type does not point to an object.
The nullptr keyword is equivalent to Nothing in Visual Basic and null in C#.
No comments:
Post a Comment