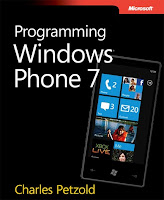
The eBook Programming Windows Phone 7, by Charles Petzold is free to download from Microsoft. It's available in PDF, ePub and mobi format.
Details:
http://blogs.msdn.com/b/microsoft_press/archive/2010/10/28/free-ebook-programming-windows-phone-7-by-charles-petzold.aspxTABLE OF CONTENTSPart I The Basics1 Hello, Windows Phone 7Targeting Windows Phone 7
The Hardware Chassis
Sensors and Services
File | New | Project
A First Silverlight Phone Program
The Standard Silverlight Files
Color Themes
Points and Pixels
The XAP is a ZIP
An XNA Program for the Phone
2 Getting OrientedSilverlight and Dynamic Layout
Orientation Events
XNA Orientation
Simple Clocks (Very Simple Clocks)
3 An Introduction to TouchLow-Level Touch Handling in XNA
The XNA Gesture Interface
Low-Level Touch Events in Silverlight
The Manipulation Events
Routed Events
Some Odd Behavior?
4 Bitmaps, Also Known as TexturesXNA Texture Drawing
The Silverlight Image Element
Images Via the Web
Image and ImageSource
Loading Local Bitmaps from Code
Capturing from the Camera
The Phone’s Photo Library
5 Sensors and ServicesAccelerometer
A Simple Bubble Level
Geographic Location
Using a Map Service
6 Issues in Application ArchitectureBasic Navigation
Passing Data to Pages
Sharing Data Among Pages
Retaining Data across Instances
The Multitasking Ideal
Task Switching on the Phone
Page State
Isolated Storage
XNA Tombstoning and Settings
Testing and Experimentation
Part II Silverlight7 XAML Power and LimitationsA TextBlock in Code
Property Inheritance
Property-Element Syntax
Colors and Brushes
Content and Content Properties
The Resources Collection
Sharing Brushes
x:Key and x:Name
An Introduction to Styles
Style Inheritance
Themes
Gradient Accents
8 Elements and PropertiesBasic Shapes
Transforms
Animating at the Speed of Video
Handling Manipulation Events
The Border Element
TextBlock Properties and Inlines
More on Images
Playing Movies
Modes of Opacity
Non-Tiled Tile Brushes
9 The Intricacies of LayoutThe Single-Cell Grid
The StackPanel Stack
Text Concatenation with StackPanel
Nested Panels
Visibility and Layout
Two ScrollViewer Applications
The Mechanism of Layout
Inside the Panel
A Single-Cell Grid Clone
A Custom Vertical StackPanel
The Retro Canvas
Canvas and ZIndex
The Canvas and Touch
The Mighty Grid
10 The App Bar and ControlsApplicationBar Icons
Jot and Application Settings
Jot and Touch
Jot and the ApplicationBar
Elements and Controls
RangeBase and Slider
The Basic Button
The Concept of Content
Theme Styles and Precedence
The Button Hierarchy
Toggling a Stopwatch
Buttons and Styles
TextBox and Keyboard Input
11 Dependency PropertiesThe Problem Illustrated
The Dependency Property Difference
Deriving from UserControl
A New Type of Toggle
Panels with Properties
Attached Properties
12 Data BindingsSource and Target
Target and Mode
Binding Converters
Relative Source
The “this” Source
Notification Mechanisms
A Simple Binding Server
Setting the DataContext
Simple Decision Making
Converters with Properties
Give and Take
TextBox Binding Updates
13 Vector GraphicsThe Shapes Library
Canvas and Grid
Overlapping and ZIndex
Polylines and Custom Curves
Caps, Joins, and Dashes
Polygon and Fill
The Stretch Property
Dynamic Polygons
The Path Element
Geometries and Transforms
Grouping Geometries
The Versatile PathGeometry
The ArcSegment
Bézier Curves
The Path Markup Syntax
How This Chapter Was Created
14 Raster GraphicsThe Bitmap Class Hierarchy
WriteableBitmap and UIElement
The Pixel Bits
Vector Graphics on a Bitmap
Images and Tombstoning
Saving to the Picture Library
Becoming a Photo Extras Application
15 AnimationsFrame-Based vs. Time-Based
Animation Targets
Click and Spin
Some Variations
XAML-Based Animations
A Cautionary Tale
Key Frame Animations
Trigger on Loaded
Animating Attached Properties (or Not)
Splines and Key Frames
The Bouncing Ball Problem
The Easing Functions
Animating Perspective Transforms
Animations and Property Precedence
16 The Two TemplatesContentControl and DataTemplate
Examining the Visual Tree
ControlTemplate Basics
The Visual State Manager
Sharing and Reusing Styles and Templates
Custom Controls in a Library
Variations on the Slider
The Ever-Handy Thumb
Custom Controls
17 Items ControlsItems Controls and Visual Trees
Customizing Item Displays
ListBox Selection
Binding to ItemsSource
Databases and Business Objects
Fun with DataTemplates
Sorting
Changing the Panel
The DataTemplate Bar Chart
A Card File Metaphor
18 Pivot and PanoramaCompare and Contrast
Music by Composer
The XNA Connection
The XNA Music Classes: MediaLibrary
Displaying the Albums
The XNA Music Classes: MediaPlayer
Part III XNA19 Principles of MovementThe Naïve Approach
A Brief Review of Vectors
Moving Sprites with Vectors
Working with Parametric Equations
Fiddling with the Transfer Function
Scaling the Text
Two Text Rotation Programs
20 Textures and SpritesThe Draw Variants
Another Hello Program?
Driving Around the Block
Movement Along a Polyline
The Elliptical Course
A Generalized Curve Solution
21 Dynamic TexturesThe Render Target
Preserving Render Target Contents
Drawing Lines
Manipulating the Pixel Bits
The Geometry of Line Drawing
Modifying Existing Images
22 From Gestures to TransformsGestures and Properties
Scale and Rotate
Matrix Transforms
The Pinch Gesture
Flick and Inertia
The Mandelbrot Set
Pan and Zoom
Game Components
Affine and Non-Affine Transforms
23 Touch and PlayMore Game Components
The PhingerPaint Canvas
A Little Tour Through SpinPaint
The SpinPaint Code
The Actual Drawing
PhreeCell and a Deck of Cards
The Playing Field
Play and Replay
24 Tilt and Play3D Vectors
A Better Bubble Visualization
The Graphical Rendition
Follow the Rolling Ball
Navigating a Maze